The blockchain ecosystem continues to fragment across multiple chains, creating a need for seamless cross-chain asset movement. Wallets that can natively handle cross-chain swaps provide significant value to users.
In this guide, I’ll walk through the essential steps to build a blockchain wallet with integrated cross-chain swap functionality using LI.FI powerful infrastructure.
Why Add Cross-Chain Swaps To Your Wallet?
Before going into implementation, let’s understand why this feature matters:
- Improved UX: Users can perform complex cross-chain operations without leaving your wallet
- Increased retention: Fewer reasons for users to seek other solutions
- New revenue streams: Potential to earn fees from swap transactions
- Competitive advantage: Differentiate your wallet from the growing competition
Core Technical Components
To build a wallet with cross-chain capabilities, you’ll need:
- Base wallet functionality: Key management, transaction signing, balance display
- Cross-chain swap integration: A bridge aggregation layer like LI.FI
- Gas management: Handling gas fees across different chains
- UI/UX for cross-chain operations: Making complex operations intuitive
Implementation Steps
Here are 7 implementation steps you need to do:
1. Set Up Basic Wallet Infrastructure
First, establish the foundational components:
// Example wallet initialization
class BlockchainWallet {
constructor() {
this.accounts = [];
this.networks = [];
this.currentNetwork = null;
}
async createAccount() {
// Generate keypair, store securely
}
async signTransaction(tx) {
// Handle transaction signing
}
}
2. Integrate LI.FI SDK
LI.FI provides a comprehensive SDK that handles the complexities of cross-chain operations:
import { LiFi } from ‘@lifi/sdk’;
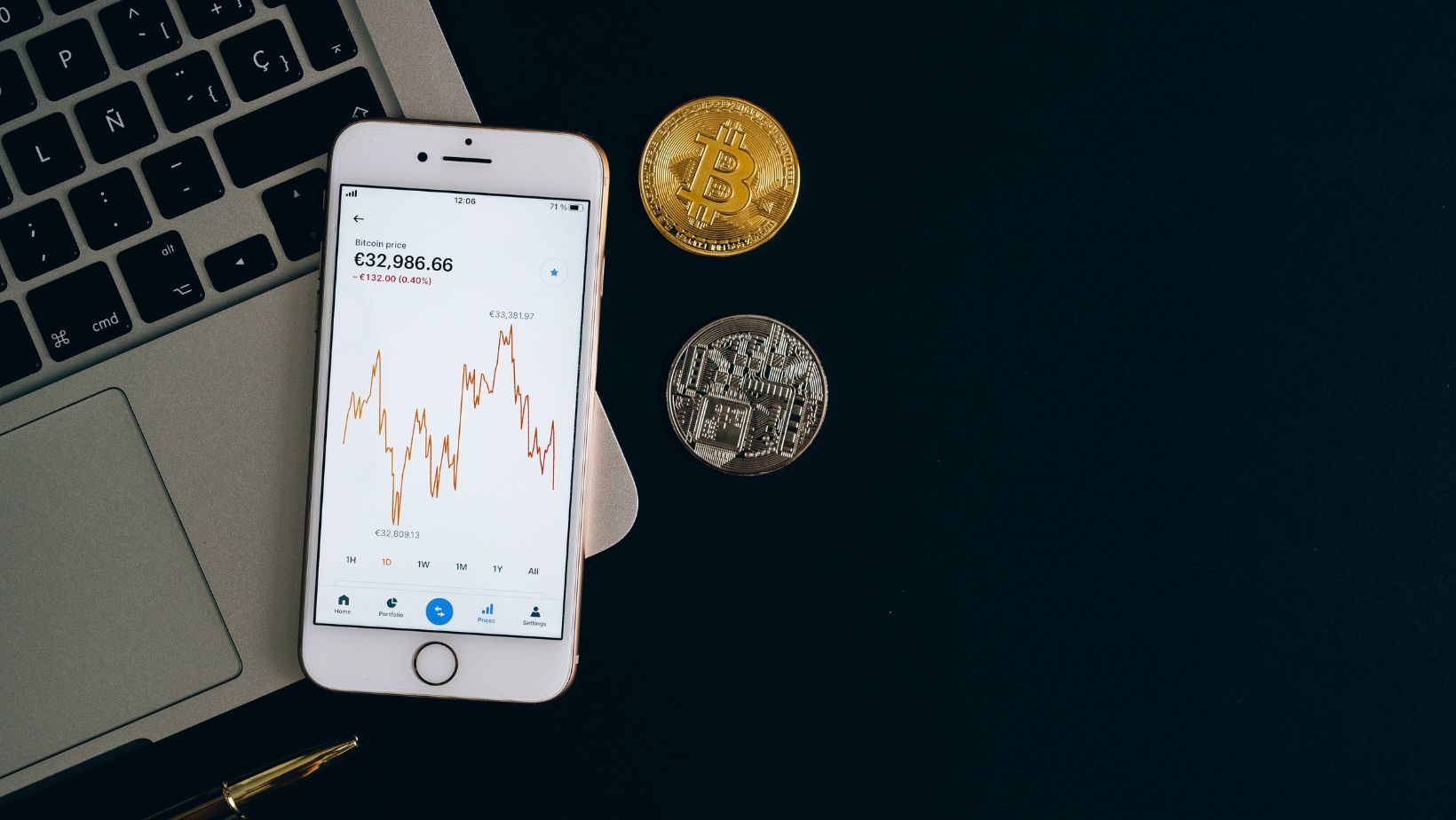
class CrossChainWallet extends BlockchainWallet {
constructor() {
super();
this.lifi = new LiFi({
integrator: ‘YOUR_WALLET_NAME’
});
}
async getRoutes(params) {
const routes = await this.lifi.getRoutes(params);
return routes;
}
async executeRoute(route) {
// Execute the selected route
return await this.lifi.executeRoute(route);
}
}
3. Implement Chain Switching
Users need to easily switch between chains:
async switchChain(chainId) {
// Validate chain is supported
if (!this.networks.includes(chainId)) {
throw new Error(‘Chain not supported’);
}
// Update current network
this.currentNetwork = chainId;
// Emit chain changed event
this.emit(‘chainChanged’, chainId);
}
4. Create a Gas Management System
Cross-chain transactions require gas on both source and destination chains:
async estimateGasCosts(route) {
// Fetch gas prices for source chain
const sourceGasPrice = await this.getGasPrice(route.fromChain);
// Calculate estimated gas for transaction
const estimatedGas = route.steps.reduce((total, step) => {
return total + step.estimatedGas;
}, 0);
return estimatedGas * sourceGasPrice;
}
5. Build the Cross-chain Swap UI
Design an intuitive interface that guides users through the swap process:
function CrossChainSwapInterface({ wallet }) {
const [fromToken, setFromToken] = useState(null);
const [toToken, setToToken] = useState(null);
const [amount, setAmount] = useState(‘0’);
const [routes, setRoutes] = useState([]);
async function findRoutes() {
if (!fromToken || !toToken || !amount) return;
const routesResult = await wallet.getRoutes({
fromChain: fromToken.chainId,
toChain: toToken.chainId,
fromToken: fromToken.address,
toToken: toToken.address,
fromAmount: amount,
});
setRoutes(routesResult.routes);
}
return (
// Render your swap interface
);
}
6. Implement Route Selection and Execution
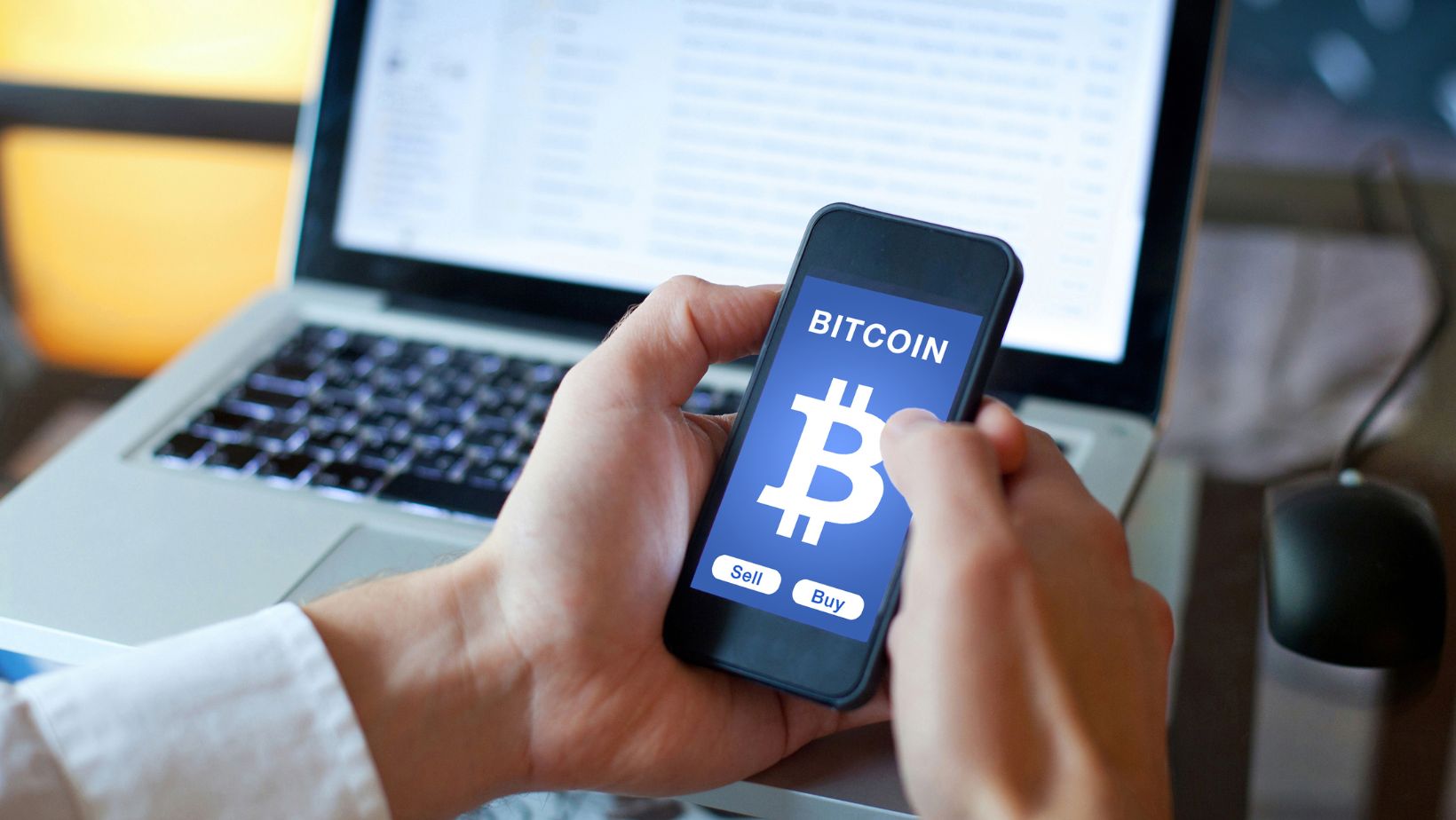
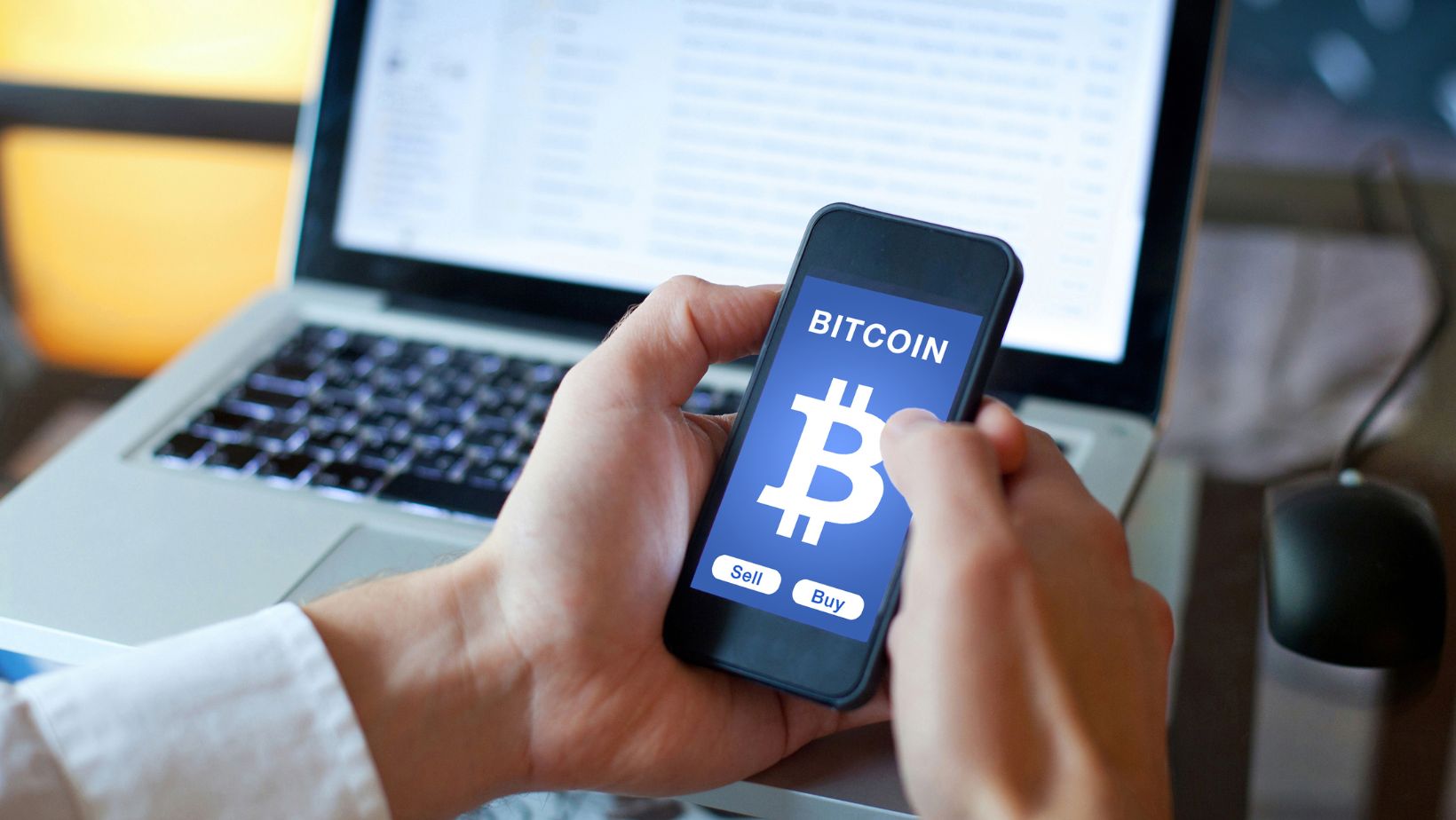
Allow users to compare and select the best route for their swap:
function RouteSelector({ routes, onSelectRoute }) {
return (
<div className=”routes-container”>
{routes.map(route => (
<RouteCard
key={route.id}
route={route}
onClick={() => onSelectRoute(route)}
/>
))}
</div>
);
}
function RouteCard({ route, onClick }) {
return (
<div className=”route-card” onClick={onClick}>
<div>Gas Fee: {route.gasCost}</div>
<div>Duration: {route.estimatedDuration}s</div>
<div>You Receive: {route.toAmount} {route.toToken.symbol}</div>
</div>
);
}
7. Add Transaction Tracking
Keep users informed about their swap progress:
function SwapProgressTracker({ execution }) {
const [status, setStatus] = useState(execution.status);
useEffect(() => {
const handleStatusUpdate = (update) => {
setStatus(update.status);
};
execution.on(‘statusUpdate’, handleStatusUpdate);
return () => execution.off(‘statusUpdate’, handleStatusUpdate);
}, [execution]);
return (
<div className=”progress-tracker”>
<StatusIndicator status={status} />
<TransactionSteps steps={execution.steps} />
</div>
);
}
Integration With Existing Wallets
For those who already possess a wallet and are seeking to infuse it with a cross-chain module, LI.FI unveils solutions that are effortlessly intermingled. For example, the recent integration with MetaMask Bridges demonstrates how existing wallets can quickly expand their capabilities.
The process of crafting a blockchain wallet, one that’s engineered with cross-chain swaps in mind, has been simplified to an impressive degree. Mostly, thanks to infrastructure providers like LI.FI. By following this guide, you’ll be able to create a wallet that meets the growing demand for seamless cross-chain experiences.